There's been a lot of talk lately about AWS Amplify, but why? , what is it? and what is it for?
AWS Amplify is a set of tools (open source framework, administration user interface, console) and services (static web hosting) that can be used together or individually to be a full stack option for creating a project.
Some of the web and mobile integration options include: Javascript, React, React Native, Angular, Vue, Next.js, Android, iOS, Ionic and Flutter. For some Frameworks, we have what they call UI Components that provide us with useful components for faster development.
However, it can become overwhelming the amount of documentation and options we have.
Today we are going to focus on an application with aws amplify that will consist of creating an application in React, using Amplify to add an authorization with AWS Cognito, creating an API (API GATEWAY) with different paths and connecting it to lambda functions, in this way we have a structure, which according to your need or imagination can serve as a template for infinite projects.
😉 Prerequisites:
- Node.js v12.x or later
- npm v5.x or later
- Git v2.14.1 or later
- Amplify cli
Once you're logged in, Amplify CLI will ask you to create an IAM user. If you already have a user created, you can return to the terminal, press enter and add your credentials.
Example:
🚀 Now it's time to start with the project:
We are going to go step by step, some steps have one or more ways to implement, you can follow the option that best suits you.
🧠 Step 1: Create React App
🧠 Step 2: Start Amplify
- Fact: Amplify has a series of very complete commands that will help us to do everything we need from our terminal.
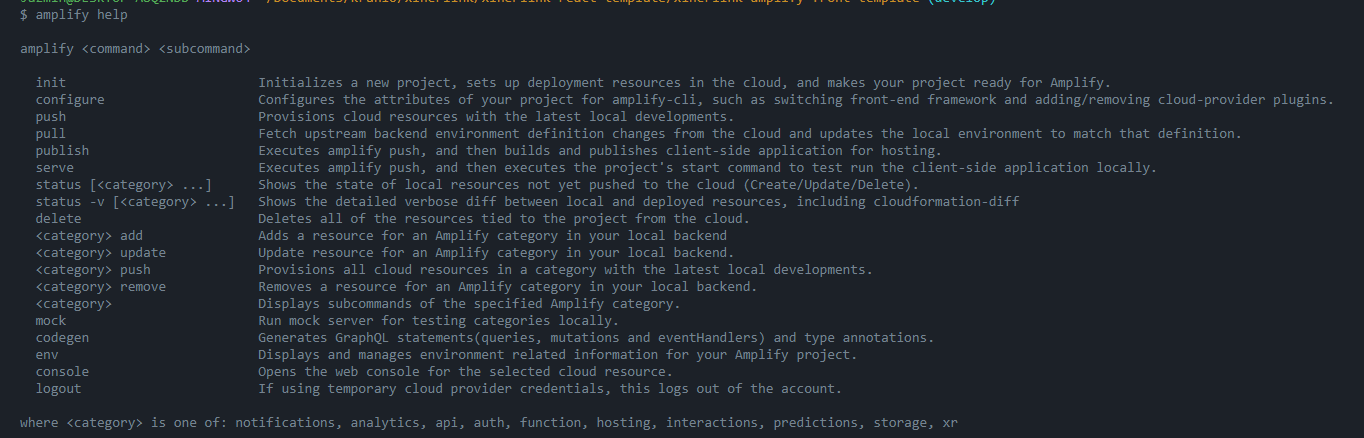
In the following code block you can see an example of how to start Amplify in your React App:
MORE INFO: https://docs.amplify.aws/start/getting-started/setup/q/integration/react/#initialize-a-new-backend
🧠 Step 3: Install amplify libraries (React)
🧠 Step 4: Configure Frontend
The file aws-exports is a configuration file generated by ampify.
🧠 Step 5: Create or import Auth (Cognito) to Amplify
If you don't have an existing Cognito you can create one like this:
Import an existing Cognito:
MORE INFO: https://docs.amplify.aws/cli/auth/import/
🧠 Step 6: Use auth to login on the front-end
To use this authorization (created or imported) in our App, we have two ways:
- With WithAuthenticator:
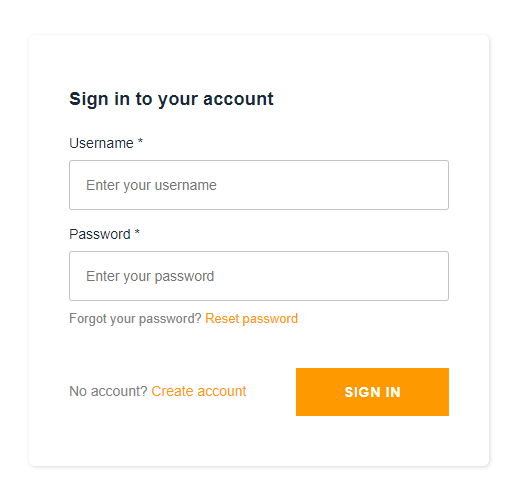
🔹 Now to access your app you must create an account (cognito), for that you can use the option create account, confirm with your email and log in.
🔹 But you also have the option of accessing Cognito from the aws console, searching for your UserPool and adding users manually:

If it is a private application, it is recommended to disable the option. Create account and only create accounts from the console, so anyone will not sign in or be able to create accounts.
🔹 To disable create account. In the section Policies answer the second question with the option: Allow only administrators to create users.
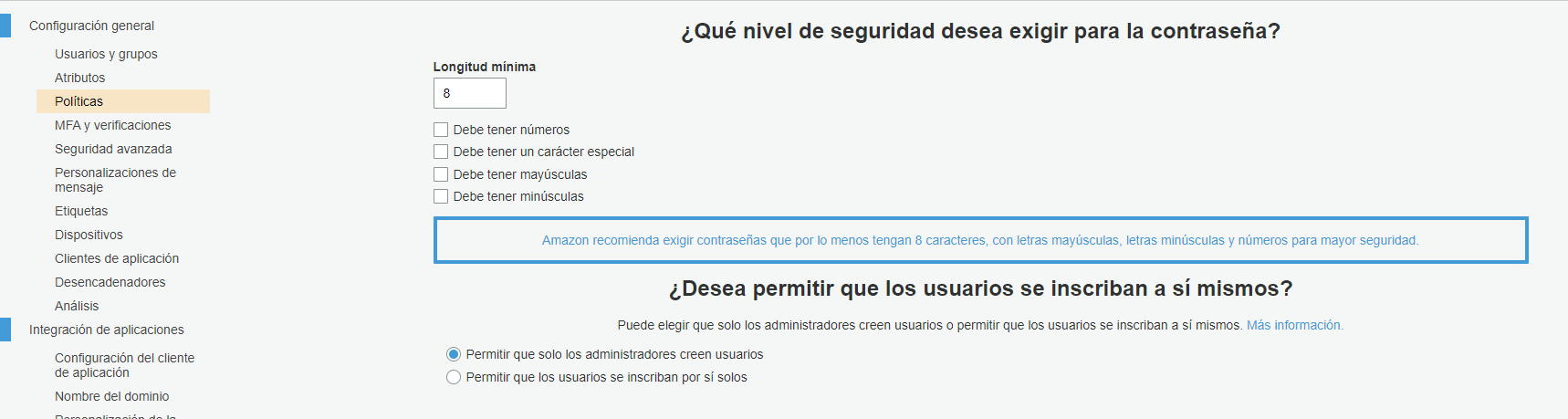
- With Customization:
We have the option of customizing our Login, for that we need knowledge about the library that is being used, in this case React. You can get an idea with this documentation:
https://docs.amplify.aws/lib/auth/emailpassword/q/platform/js/#custom-attributes
https://docs.amplify.aws/start/getting-started/auth/q/integration/react/#create-login-ui
🧠 Step 7: Create an API Gateway with amplify (REST)
When creating an API we have many possibilities, today we will see how to add two paths linked each to a different lambda function.
Before creating the API, let's understand what we want to achieve. We're going to join lambda functions to different paths in our API.
In this way:
When creating our API we will have to create a path immediately, so we are going to add one in this step and then we will see how to add another one.
- When adding a function, it gives us three options such as “template”, each one has different purposes:
MORE INFO: https://docs.amplify.aws/cli/function/#set-up-a-function
Once the API is created, we can import it and configure it in our App.
We also have the option of importing an existing API just by omitting the creation and adding it to the configuration directly.
This configuration allows us to make direct requests to the API, for example for a GET:
🧠 Step 8: Add another path to our API
In the previous step we created our API and configured it, now we are going to add a new path and we are going to join it to a new Lambda function just like the previous path.
These actions generated folders with the resources inside the Amplify/BackEnd directory, where we can work on the functionality for the lambdas.
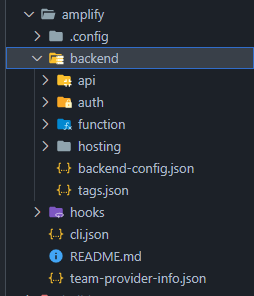
🧠 Step 9: Amplify Deploy
After creating our resources in amplify, both the frontend and the backend, we need to implement them. For this we have several possibilities.
We have the command:
To implement only the backend of our amplify project.
We also have the command:
Which will apply both the frontend and the backend of our project. But to implement the frontend, first we have to create a hosting which consists of a structure that will house the web application, we have three: amplify console, directly in an S3 bucket or a combination of an S3 bucket with Cloudfront.
For this we use the command:
MORE INFO: https://docs.amplify.aws/cli/hosting/hosting/
Amplify CI/CD
We have two possibilities to have a continuous deployment pipeline with amplify: manual or automated by amplify. When doing an add hosting you can choose how you want to do it, the interesting thing is that you can have a specific type for each environment and a format in dev, a format in a feature branch, a format for qa, always choosing what best suits you.
The following is an example of an automated Amplify deployment:
- We first located the front environment of our App in AWS Amplify and connected it to our repository:
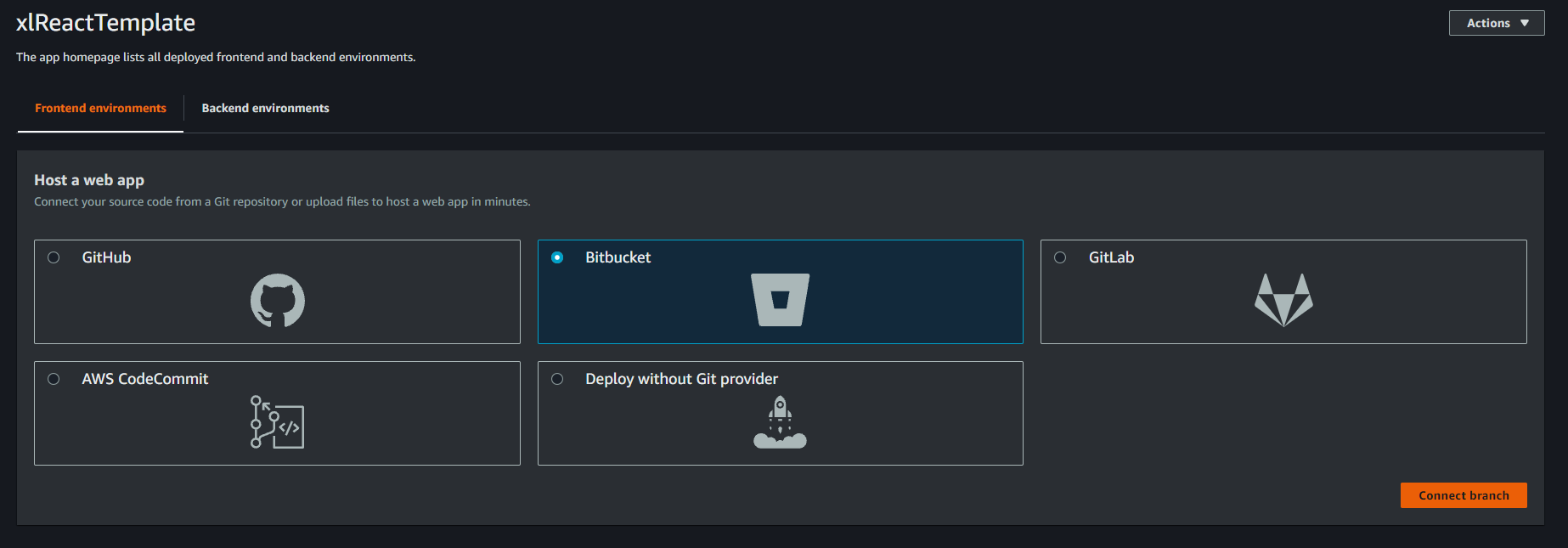
- Then we choose the branch where we want to deploy, in this case Develop, however, as mentioned above, you can configure as many automated deployments as there are branches in our project.
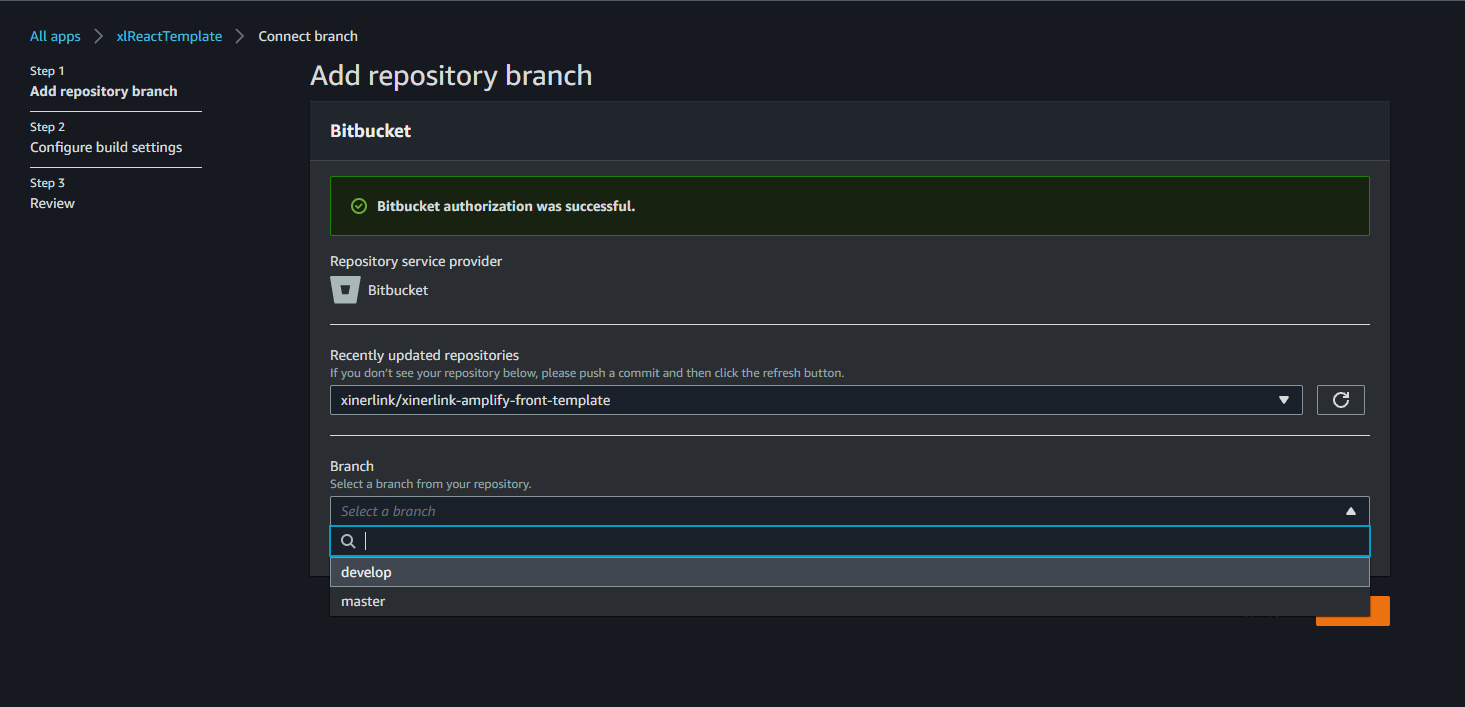
- The next thing is to select our application in the environment (dev) and add a role with access to amplify. If there is none you can easily create in the Create new role option and follow the steps indicated, then refreshing so that it appears is enough.

- This is necessary to continue:
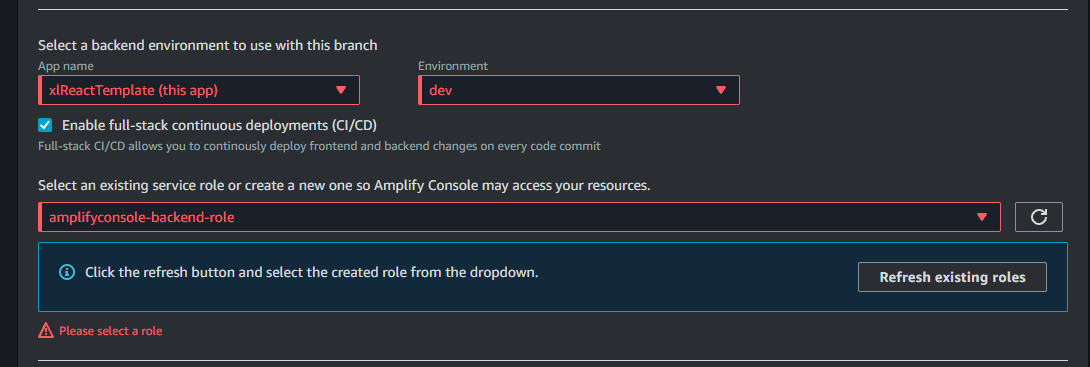
- Before proceeding to the next step, click on the box: Allow AWS Amplify to automatically deploy all files hosted in your project root directory
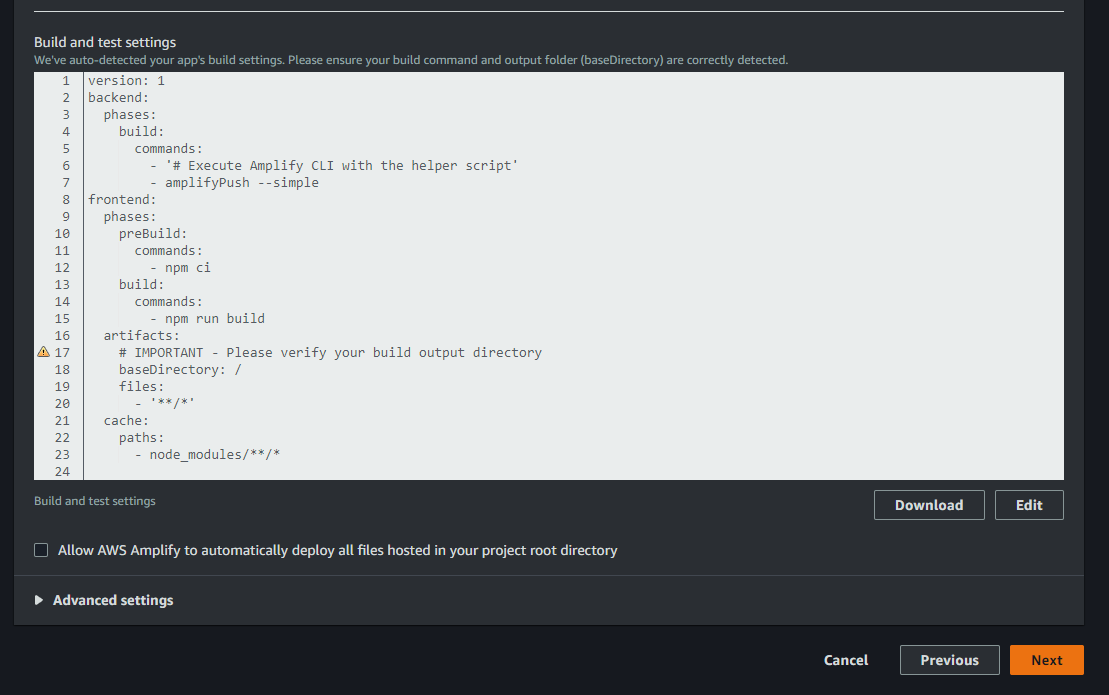
- Finally We Push Save and Deploy And that's all, the application will start deploying:

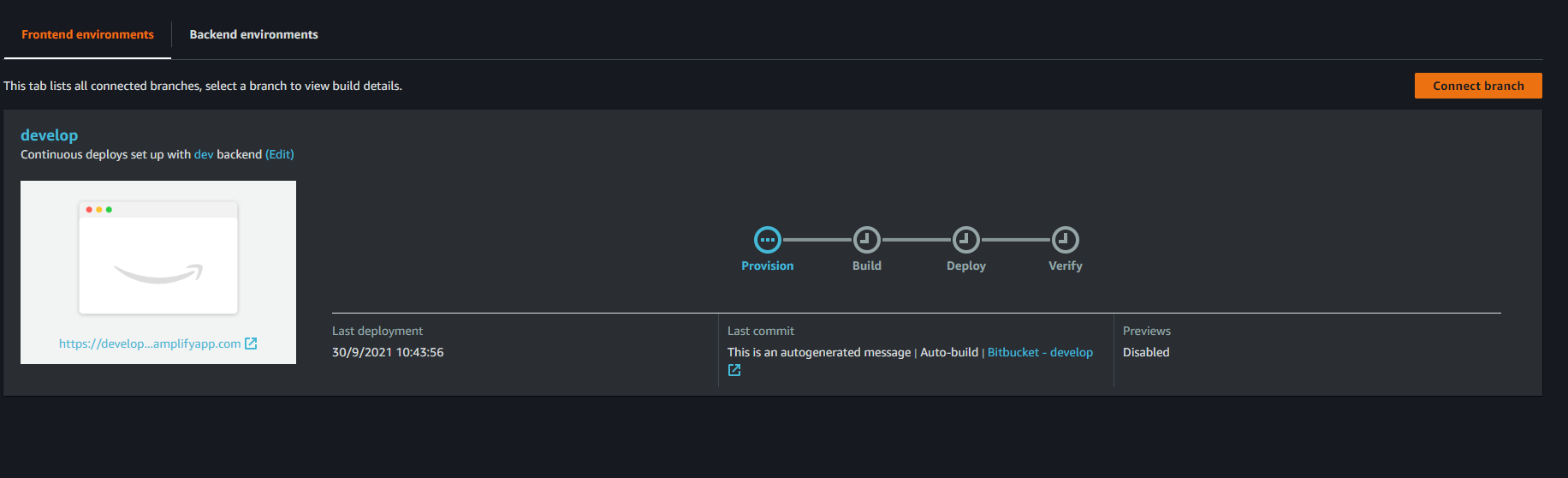
🧠 Conclusion
Finally, now you know what amplify is, its options, and you have an example guide on how to implement Backend services such as API Gateway, Cognito, Lambda Functions with a specific Front-End library. You have a process for creating an application with aws amplify. It's time to adapt it to your needs. Whether you have a front application that needs a fast and scalable backend or if you want to start from scratch, this will undoubtedly be a good option.
Do you have an idea? Don't you know how to start? Write to us.
Co-author: Gustavo Matozinho