introduction
In modern web design, CSS animations have emerged as a fundamental tool for improving the interactivity and aesthetics of web pages. These animations allow developers to implement smooth transitions and dynamic visual effects that not only capture the user's attention, but also improve the usability and overall experience of the site. Throughout this document, we'll explore the fundamentals of CSS animations, from the animation property to the rules @keyframes
, and we'll discuss how they can be applied to create more attractive and functional interfaces.
CSS animations
CSS animations allow designers to create smooth transitions and eye-catching visual effects relatively easily. When using properties such as Animation-name, animation-duration, animation-timing-function, and more, you can meticulously control how the elements on the page come to life.
Animation property:The property Animation CSS is a shortened form that combines several sub-properties that control different aspects of an animation:
Animation-name
: Define the name of the @keyframes which controls the animation.animation-duration
: Set how long an animation cycle lasts.animation-timing-function
: Controls the speed curve of the animation (for example, Linear, Ease-In, Ease-Out, Ease-in-Out).Animation-Delay
: Set a waiting time before the animation starts.animation-iteration-count
: Defines how many times the animation is repeated (it can be a number or Infinite for an infinite loop).Animation-fill-mode
: Specifies a style for the element when the animation is not playing (for example, before starting, after finishing).
Rule @keyframes:This rule defines the states of the animation, specifying the styles at various points over time. You can define from two points (start and end) to multiple intermediate points:
How They Work
When you apply an animation to an element, the browser interpolates the styles between the points defined in the @keyframes
. This means that it calculates the intermediate values between the points you specified. For example, if you animate the background-color
from red to green, the browser automatically generates intermediate colors during the course of the animation.
Examples:
- Simple Animation: A button that changes color gradually as you move the cursor.
- Complex Animation: Blue box with a scrolling animation

Skeleton as a more elaborate example
The “skeleton” effect is commonly used in interface design to improve the user experience when loading content. These “skeleton” elements are often animated to indicate that the content is in the process of loading, and animations such as pulsations or soft movements are often used to attract attention and improve the perception of performance.
I'm going to adapt the previous example to create a pulsation effect that could be applied to a skeleton element while waiting for the content to load.

To view the full code and explore how it was implemented, you can visit the following link to the repository. There you will find all the necessary details to appreciate the implementation and functionality of the animation: https://github.com/karlacabanas01/skeleton
Pseudoclasses in Animations
Pseudoclasses determine the state of an element to which an animation is applied, allowing animations based on user interaction to be activated. Some examples include:
:hover
: Starts an animation when the user hovers the cursor over an element.:focus
: Activate an animation when an element gains focus, commonly used in forms.:active
: Trigger an animation during the interaction, such as when clicking a button.:checked
: Apply animations to selected form elements to improve visual response.
Pseudo-elements in Animations
Pseudoelements allow you to animate specific parts of an element without adding more elements to the HTML, ideal for adding aesthetic details or visual effects.
::before
and::after
: They are used to create additional content before or after the main content of an element. These pseudo-elements can be animated to create effects such as load animations, decorations that appear or transform in response to an interaction.
⚠️ Key Differences:
- Pseudoclasses: They apply to specific states or conditions of an element, such as
:hover
or:active
. - Pseudo-elements: They allow you to style specific parts of an element, acting as virtual “sub-elements”, examples include
::before
and::after
Comparison table between transitions and animations in CSS:Transformations:
%2011.37.16%E2%80%AFp.%C2%A0m..png)
- Animation-Direction: Indicates if the animation should alternate its direction (go and return).
%2011.23.58%E2%80%AFp.%C2%A0m..png)
Las transformations CSS allows you to modify the spatial appearance of an element, such as its size, shape, position and orientation, without affecting other elements in the document. The transformations do not alter the flow of the document, that is, the original space of the element remains the same. Transformations are powerful for creating dynamic visual effects.
Most common transformation properties:
- Translate (x, y): Move an element in the horizontal (x) and vertical (y) plane.
- scale (left, sy): Scale an element, increasing or decreasing its size. If only specified
SX
, the scaling will be uniform. - rotate (angle): Rotate an element around its center point.
- SkewX (angle) and SkeWy (angle): Tilts an element by distorting its X or Y axes.
Transitions:
%2011.22.06%E2%80%AFp.%C2%A0m..png)
Transitions are ideal for subtle effects, such as changing the background color of a button when you hover the cursor.
Las transitions provide a way to control the change of CSS property values over a period of time. This is especially useful for animating state changes, such as en:hover or when modifying properties using JavaScript.
Key components of a transition:
- Transition: function-time delay duration property;
transition: background-color 0.3s ease;
- transition-property: The CSS property to be animated.
- transition-duration: How long the transition will last.
- transition-timing-function: Defines how intermediate values are calculated (for example,
Linear
,Ease-In
,Ease-Out
). - transition-delay: Waiting time before the transition begins.
Example of a transition:
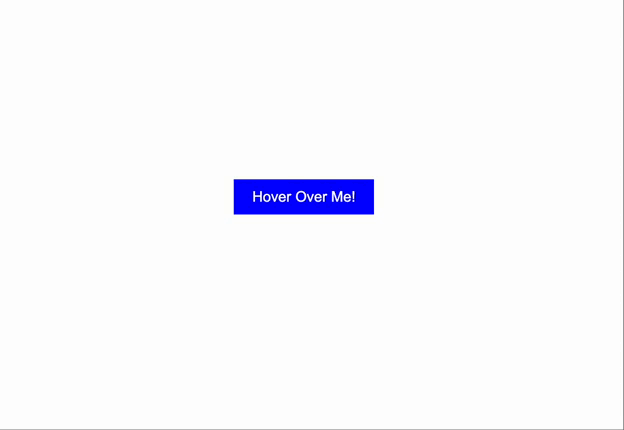
The use of Pseudoclasses in CSS for transitions:
:hover
: Activate transitions when you hover your mouse over an element.:focus
: Apply transitions when an element gains focus.:active
: Initiate transitions during interaction, such as when clicking.:checked
: Used for transitions on selected elements, such as checkboxes.:not ()
: Apply styles excluding specific elements in transitions.
And on the other hand the pseudo-elements in CSS, such as ::before
and ::after
, are used in transitions to add visual effects without modifying the HTML. You can apply transitions to these to animate decorative elements, such as lines or icons, that appear before or after the main content of an element.
☝🏻 Differences
- Transformations are used to apply geometric effects to elements. They are static if not combined with transitions or animations.
- Transitions are ideal for animating changes to CSS properties in a smooth and gradual way. They require a change in the value of the property to be activated, such as a change of state or a scripted modification.
Comparison table between transitions and animations in CSS:
%2010.29.21%E2%80%AFp.%C2%A0m..png)
Project with the concepts learned:
This project represents an initial prototype of what a basic web page might look like, including read-only elements. It also incorporates some concepts discussed in the three previous articles. In addition, I will provide a link to the project on GitHub to facilitate a more detailed and understandable view.
%2010.52.29%E2%80%AFa.%C2%A0m..png)
For a more detailed view and understanding of the code and the elements discussed in previous posts, as well as this article on CSS, visit the following link to the project repository. Here you can directly explore how these concepts have been implemented on a real website, thus facilitating a better appreciation of the learning achieved so far.
https://github.com/karlacabanas01/page-post-3
Conclusion
CSS animations are an incredibly powerful tool in any front-end developer's arsenal, providing a means to not only attract users but also to guide their interaction through the user interface in an intuitive way. By integrating techniques such as transformations, transitions, and detailed animations, developers can create a richer and more engaging user experience. In addition, by using pseudoclasses and pseudo-elements to control these animations, a range of possibilities opens up to designing interfaces that are not only beautiful, but also highly functional and responsive to user actions. As we continue to explore and experiment with these techniques, we're sure to see even more creative innovations in web design in the future.
Ready to take your website's user experience to the next level with effective CSS animations?
At Kranio, we have experts in web design and development who will help you implement CSS animations that improve the interactivity and aesthetics of your pages, ensuring an optimal user experience. Contact us and discover how we can help you create more attractive and functional interfaces.